Basics of Python Programming
Basic topics you will learn here are ๐๐
Features of Python:
1. Unsophisticated :
It is an unsophisticated language. It allows the user to write the programs without following the strict rules viz., declaring the variable data types, usage of curly braces etc. It allows the programmer to provide the solution program rather than focusing on the structure and syntax.
2. Easy :
3. High-level :
The programmer need not worry about various low-level details required for the program. Python is understood as a high-level, interpreted general-purpose language. This means it is not your straight compiled language (like Java or C) but an interpreted dynamic language that has to be run in the given system using another program instead of its local processor.
4. Portable :
The Python is a platform independent programming language. With the Byte code, the Python Program can be executed by any platform and hence the Python programs are portable.
5. Interpreted :
The Python programs can be directly executed from the Byte code. The Byte code is an executable code but it is an interpreted code. It translates the original source code into platform independent code.
6. Object Oriented :
Python supports all the principles of object-oriented programming. The Python programs can be designed around the objects which concentrate on the data. Implementing various OOPs principles in Python is simple than compared to C++ or Java.
7 . Extensibility :
These programs are extensible to C, C++ and Java. These programs can be executed by the Python. In this case, the programs can be treated as disabled codes.
8. Embeddable :
9. Extensive Libraries :
The Python consists of huge standard library for handling regular expressions, threading, GUI etc
![]() |
Features of python |
Merits & Demerits :
![]() |
Merits and Demerits |
Writing and Executing First Python Program:
When programming in Python, you have two basic options for running code : interactive mode and script mode .
Interactive Mode:
Interactive mode is great for quickly and conveniently running single lines or blocks of code. Here's an example using the python shell that comes with a basic python installation. The “>>>” indicates that the shell is ready to accept interactive commands. Open the Python Interactive Mode.
Enter the following code and press Enter, the message “Hello World” will display in the next line.
Script Mode:
If instead you are working with more than a few lines of code, or you‟re ready to write an actual program, script mode is what you need. Instead of having to run one line or block of code at a time, you can type up all your code in one text file, or script, and run all the code at once.
Constants:
A constant is a type of variable whose value cannot be changed. It is helpful to think of constants as containers that hold information which cannot be changed later.
Literals :
Literal is a raw data given in a variable or constant. In Python, there are various types of literals they are as follows:
- Numeric Literals
- String Literals
- Boolean Literals
- Special Literals
- Literal Collections
Numeric Literals:
Numeric Literals are immutable (unchangeable). Numeric literals can belong to 3 different numerical types: Integer, Float, and Complex.
a = 0b1010 #Binary Literals
b = 100 #Decimal Literal
c = 0o310 #Octal Literal
d = 0x12c #Hexadecimal Literal
#Float Literal
float_1 = 10.5
float_2 = 1.5e2
#Complex Literal
x = 3.14j
print(a, b, c, d)
print(float_1, float_2)
print(x, x.imag, x.real)
String Literals :
A string literal is a sequence of characters surrounded by quotes. We can use both single, double, or triple quotes for a string. And, a character literal is a single character surrounded by single or double quotes. strings = "This is Python"
Boolean Literals :
A Boolean literal can have any of the two values: True or False.
y = (1 == False)
a = True + 4
b = False + 10
print("x is", x)
print("y is", y)
print("a:", a)
print("b:", b)
Special Literals :
Python contains one special literal i.e. None. We use it to specify that the field has not been created.
Literal Collections :
There are four different literal collections List literals, Tuple literals, Dict literals, and Set literals.
Variables :
In Python, variables can be initialized without declaration. Based on the value of the input data, the type of the variable will be automatically considered. A variable name should start with a letter followed by sequence of letters, numbers or underscore (_) special character. Integer data, floating point data and string data will be considered as primitive data type. Character data will be considered as string data with length 1.
In Python, the string data will be considered as an array. The array index will start from 0.
>>> a
'chapter-2'
>>> a[1]
'h'
Tuples:
It is a list with a fixed number of elements. It uses parentheses for specification of data.
Dictionaries:
Identifiers :
- The identifier can be a combination of various elements viz., uppercase letters, lowercase letters, digits and underscore.
- Any one of the keyword is not permitted to act as identifier.
- The first letter of the identifier should not be a digit.
- Special alphabets viz., !,@,# etc… are also not permitted to be a part of identifier.
Data Types:
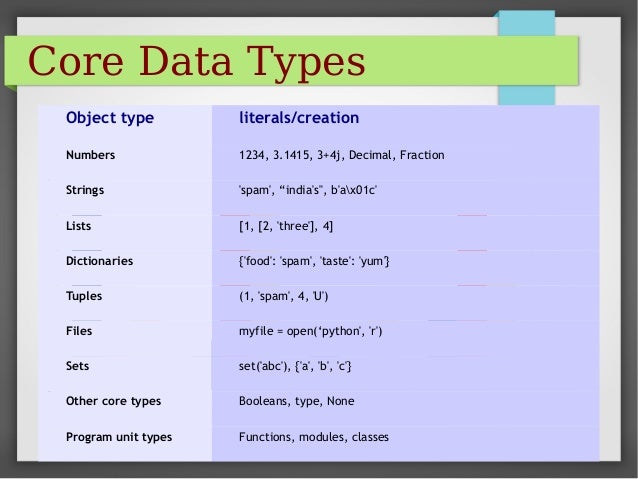
- If the value is “None” .
- If the value is “0” or “0.0” .
- If the input value is empty list, tuple or string.
Input & Output Operation:
Comments:
- “#” is used to represent the single line comment.
- Multiple line comment statements are started and ended with the ''' ''' delimeter.
Indentation:
In Python programming language, group of code or statements are arranged in an individual block. Each block can be separated with the indentation.
Block1
Block2
Block3
Block2
Block1
Structure blocks in Python with indentation๐๐
Operators :
The Python programming language supports the following operators.
![]() |
Operators |
Arithmetic Operators :
The arithmetic operators are used to perform the arithmetic operations between any two operands. Various arithmetic operators are
- The „+‟ operator is used to perform the addition operation between the two input data values.
- The „-‟ operator is used to perform the subtraction operation between the two input data values.
- The „*‟ operator is used to perform the multiplication operation between the two input data values.
- The „/‟ operator is used to perform the division operation between the two input data values.
- The „%‟ operator is used to return the remainder of the division operation performed between the two input data values.
- The „**‟ operator is used to compute the exponential calculation.
- The „//‟ operator is used to perform the floor division operation between the two input data values. It returns the quotient of the division operation. The decimal points in the quotient result will be removed. If any one of the input is negative then the quotient result is rounded towards negative infinity. For example the two numbers considered are 20 and 10 then the result of
a-b = 10
a+b = 30
a*b = 200
a/b = 2
a%b = 0 (remainder is 0)
a**2 = 400 (202)-a//b = -2 (a is negative, so the quotient will be rounded to towards negative infinity)
Comparison (Relational) Operators :
These operators are used to estimate the relation between the given two input values. Various relational operators are
- The „==‟ operator is used to compare the two given input values whether they are equal or not. It returns True when both are equal otherwise it returns False.
- The „!=‟ operator is used to compare the two given input values whether they are equal or not. It returns True when both are not equal otherwise it returns False.
- The „>‟ operator is used to compare the two given input values whether the left side value is greater than right side value or not. It returns True if the left side value is greater than the other value otherwise it returns False.
- The „<‟ operator is used to compare the two given input values whether the left side value is less than right side value or not. It returns True if the left side value is less than the other value otherwise it returns False.
- The „>=‟ operator is used to compare the two given input values whether the left side value is greater than or equal to right side value or not. It returns True if the left side value is greater than or equal to the other value otherwise it returns False.
- The „<=‟ operator is used to compare the two given input values whether the left side value is less than or equal to right side value or not. It returns True if the left side value is less than or equal to the other value otherwise it returns False.
For example the two numbers considered are 20 and 10 then the result of
(a==b) → False
(a!=b) → True
(a>b) → True
(a<b) → False
(a>=b) → True
(a<=b) → False
Assignment Operators :
The assignment operator is used to assign the input value to the variable. The assignment operator can be grouped with the arithmetic operators and are called as compound assignment operators. Various assignment operators are
- The „=‟ operator is used to assign the input value (right side value) to the variable (left side variable).
- The „+=‟ is used to add the right side value and the left side value and assigns the result to the left side variable.
- The „-=‟ is used to subtract the right side value and the left side value and assigns the result to the left side variable.
- The „*=‟ is used to multiply the right side value and the left side value and assigns the result to the left side variable.
- The „/=‟ is used to divide the right side value and the left side value and assigns the result to the left side variable.
- The „*=‟ is used to compute the exponential of the right side value and the left side value and assigns the result to the left side variable.
- The „+=‟ is used to compute the floor division of the right side value and the left side value and assigns the result to the left side variable.
The results of the following operators are
(a=20) → value of a is 20
(a=20), (a+=10) → value of a is 30
(a=20), (a-=10) → value of a is 10
(a=20), (a*=10) → value of a is 200
(a=20), (a/=10) → value of a is 2
(a=20), (a**=2) → value of a is 400
(a=20), (a//=10) → value of a is -2
Logical Operators :
The logical relation between two input values is computed by the logical operators. Various logical operators are
- The „and‟ is called as Logical AND operator. It returns True only when both the inputs are True.
- The „or‟ is called as Logical OR operator. It returns True when at least one of input is True.
- The „not‟ is called as Logical NOT operator. It returns the negativity of the input value. For example, if two input numbers a and b are 1 and 0 then the results of the following operations are
(a and b) → False
(a or b) → True
(not (a)) → False
Bitwise Operators :
- The „&‟ is called as binary AND operation. It will select the resultant bit to True only when the corresponding two input bit values are True otherwise it is False.
- The „|‟ is called as binary OR operation. It will select the resultant bit to True when at least one of the corresponding two input bit values are True otherwise it is False.
- The „^‟ is called as binary XOR operation. It will select the resultant bit to True only when the corresponding two input bit values are different otherwise it is False.
- The „~‟ is called as binary ones complement operation. It will set the resultant bit to the complemented input bit value.
- The „<<‟ is called as binary Left Shift Operation. It will left shift the left side value. The right side value will specify the required number of shift operations.
- The „>>‟ is called as binary Right Shift Operation. It will right shift the left side value. The right side value will specify the required number of shift operations.
Membership Operators :
- The „in‟ operator is used to search the input element in the sequence. If it is found then returns true otherwise returns false.
- The „not in‟ operator is used to search the input element in the sequence. If it is not found then returns true otherwise returns false
y=2
a=(y in x)
print("in operator result=",a)
b=(y not in x)
in operator result= True
Identity Operators:
- The „is‟ operator is used to return true if both objects points to the same memory location otherwise it returns false.
- The „is not‟ operator is used to return true if both objects are not pointing to the same memory location otherwise it returns false.
y=2
a=(y is x)
print("is operator result=",a)
#Output:
is operator result= False
Expressions and Order of Evaluations :
![]() |
Operator Precedence |
Comments
Post a Comment